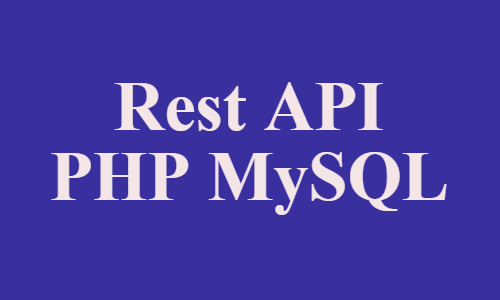
Bismillaahirrohmaanirrohiim…
Berikut ini adalah cara mudah dan cepat mengedit data menggunakan rest api update dengan PHP MySQL tanpa framework.
Ini adalah serangkaian lanjutan CRUD rest api PHP MySQL.
Untuk melanjutkan materi ini, lebih dahulu harus membaca tutorial sebelumnya tentang rest api, link ada di bawah.
Langsung saja ya,
1. Struktur file
Misalkan server anda ada di c:/xampp/htdocs/toko/
buat struktur filenya menjadi seperti dibawah ini:
api/ db/ settings.ini.php Db.class.php Log.class.php categories/ list.php create.php detail.php update.php web/ inc.php index.php kategori.php kategori-add.php kategori-edit.php
2. buat file detail.php
Untuk mengambil salah satu data dari tabel “categories” dengan rest api, kita perlu membuat file “detail.php” (lihat lokasinya di atas).
Isi file detail.php seperti di bawah ini:
<?php header('Content-Type: application/json'); include dirname(dirname(__FILE__)).'/db/Db.class.php'; $db = new Db(); $cat_id = isset($_GET['cat_id']) ? (int) $_GET['cat_id'] : 0; $cat_detail = $db->row('select * from categories where cat_id='.$cat_id); $arr = array(); $arr['info'] = 'success'; $arr['result'] = $cat_detail; echo json_encode($arr);
3. membuat file update.php
Untuk mengupdate isi dari tabel “categories” dengan rest api, kita perlu membuat file “update.php” (lihat lokasinya di atas).
Kita akan menggunakan method post, karena lebih dinamis dalam menangani parameter dan data yang banyak.
Nantinya kita akan membuat form input <form method="POST" action="API_URL">
di file kategori-edit.php
<?php header('Content-Type: application/json'); include dirname(dirname(__FILE__)).'/db/Db.class.php'; $db = new Db(); $cat_id = isset($_POST['cat_id']) ? (int) $_POST['cat_id'] : ''; $cat_name = isset($_POST['cat_name']) ? $_POST['cat_name'] : ''; $cat_description = isset($_POST['cat_description']) ? $_POST['cat_description'] : ''; if (empty($cat_id) OR empty($cat_name)) { $arr = array(); $arr['info'] = 'error'; $arr['msg'] = 'ID atau nama Kategori tidak ada'; echo json_encode($arr); exit(); } $datas = array(); $datas['cat_name'] = $cat_name; $datas['cat_description'] = $cat_description; $datas['cat_modified'] = date('Y-m-d H:i:s'); $exec = $db->update('categories', $datas,' where cat_id='.$cat_id); if (!$exec) { $arr = array(); $arr['info'] = 'error'; $arr['msg'] = 'Query tidak berhasil dijalankan.'; echo json_encode($arr); exit(); } $arr = array(); $arr['info'] = 'success'; $arr['msg'] = 'Data berhasil diproses.'; echo json_encode($arr);
4. membuat file kategori-edit.php
file ini untuk membuat form edit data dan proses pengiriman data ke API
<?php include 'inc.php'; $cat_id = isset($_GET['cat_id']) ? (int) $_GET['cat_id'] : 0; if (empty($cat_id)) { header('location:kategori.php'); exit(); } $cat_name = isset($_POST['cat_name']) ? $_POST['cat_name'] : ''; if (!empty($cat_name)) { //proses submit ke API $cat_id = isset($_POST['cat_id']) ? (int) $_POST['cat_id'] : ''; $cat_description = isset($_POST['cat_description']) ? $_POST['cat_description'] : ''; $url = $api_url.'/categories/update.php'; $postdata = array(); $postdata['cat_id'] = $cat_id; $postdata['cat_name'] = $cat_name; $postdata['cat_description'] = $cat_description; $ch = curl_init(); curl_setopt($ch, CURLOPT_URL,$url); curl_setopt($ch, CURLOPT_FOLLOWLOCATION, TRUE); curl_setopt($ch, CURLOPT_USERAGENT, "Mozilla/5.0 (Windows NT 6.1; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/37.0.2062.120 Safari/537.36"); curl_setopt($ch, CURLOPT_POST, 1); curl_setopt($ch, CURLOPT_POSTFIELDS,http_build_query($postdata,'','&')); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false); curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, false); curl_setopt($ch, CURLOPT_TIMEOUT, 30); $response = curl_exec ($ch); $http_status = curl_getinfo($ch, CURLINFO_HTTP_CODE); $curl_error = curl_error($ch); curl_close ($ch); $arr_response = json_decode($response, true); $info = isset($arr_response['info']) ? $arr_response['info'] : 'error'; $msg = isset($arr_response['msg']) ? $arr_response['msg'] : 'tidak diketahui'; header('location:kategori-edit.php?cat_id='.$cat_id.'&info='.$info.'&msg='.$msg); exit(); } ?> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <html> <head> <meta http-equiv="content-type" content="text/html; charset=utf-8"> <title>Edit Kategori</title> </head> <body> <h1>Edit Kategori</h1> <?php $info = isset($_GET['info']) ? $_GET['info'] : ''; $msg = isset($_GET['msg']) ? $_GET['msg'] : ''; if (!empty($info)) { echo 'Info: '.$info; echo '<br />Msg: '.$msg; echo '<br />'; } $api_categories_detail = $api_url.'/categories/detail.php?cat_id='.$cat_id; $json_detail = @file_get_contents($api_categories_detail); $arr_detail = json_decode($json_detail, true); $result = isset($arr_detail['result']) ? $arr_detail['result'] : array(); ?> <p><a href="kategori.php">« Back</a> | <a href="kategori-edit.php?cat_id=<?php echo $result['cat_id']; ?>">Reload</a></p> <form method="POST" action=""> <input type="hidden" name="cat_id" value="<?php echo $result['cat_id']; ?>" /> <table border="1"> <tr> <td>Nama Kategori</td> <td>:</td> <td><input type="text" name="cat_name" size="50" value="<?php echo $result['cat_name']; ?>"></td> </tr> <tr> <td>Keterangan Kategori</td> <td>:</td> <td><input type="text" name="cat_description" size="50" value="<?php echo $result['cat_description']; ?>"></td> </tr> </table> <p> <input type="submit" name="sbm" value="Submit" /> </p> </form> </body> </html>
5. Mencoba mengakses web kategori
Setelah semua file di atas siap, kita dapat mengakses alamat URL web kita, dengan cara buka dibrowser alamat URL di bawah ini:
http://localhost/toko/web/
Lalu klik link “Edit” pada salah satu data, kemudian isi nama kategori dan keterangan setelah itu di submit.
6. Selesai
Jika data yang di edit tadi muncul di dalam tabel dan isinya berubah seperti gambar di atas, berarti sudah berhasil mengupdate data menggunakan rest api di website dengan PHP MySQL.
Script di atas boleh dicopas, tapi sebaiknya diketik ulang biar lebih mudah memahaminya.
Oia, script di atas dibuat sederhana, insyaAllah banyak yang sudah memahaminya, makanya tidak perlu kami jelaskan di artikel ini.
apabila ada kode script yang belum dipahami, silahkan mengisi di kolom komentar di bawah ini.
Index Artikel Rest API dengan PHP MySQL:
1. Persiapan – Cara membuat rest api dengan php mysql mudah dan cepat
2. View – Menampilkan hasil dari rest API PHP MySQL
3. Add – Menambahkan data menggunakan rest API create PHP MySQL
4. Edit – Mengedit data menggunakan rest API PHP MySQL
5. Delete – Menghapus data menggunakan rest API PHP MySQL