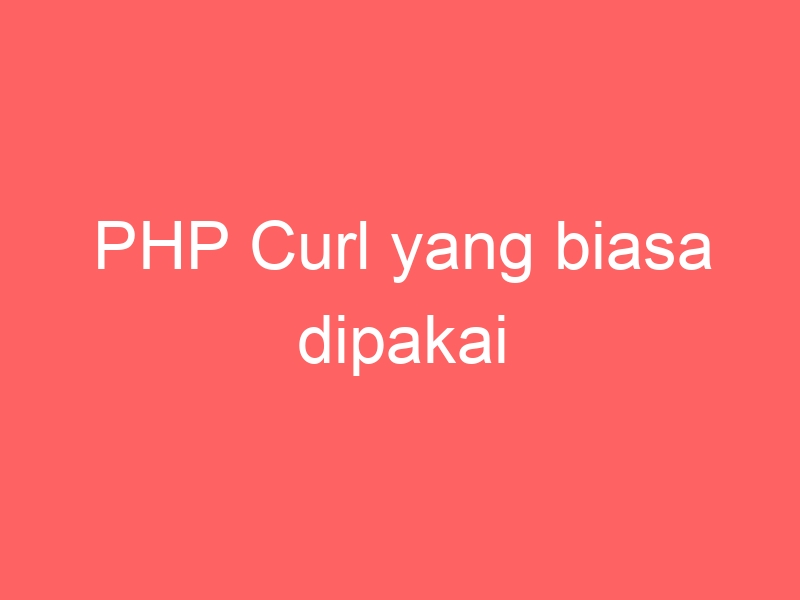
Bismillahirrohmaanirrohiim…
Posting diakhir tahun 2019 ini adalah tentang php curl yang insyaAllah akan terus diupdate jika ada perubahan versi php atau ada alasan lain.
PHP CURL post default
Kita akan mengirimkan parameter post ke server, sama seperti form method post.
$url = 'http://yourapiurl'; $postdata = array(); $postdata['param1'] = 'value 1'; $postdata['param2'] = 'value 2'; $postdata['param3'] = 'value 3'; $ch = curl_init(); curl_setopt($ch, CURLOPT_URL,$url); curl_setopt($ch, CURLOPT_FOLLOWLOCATION, TRUE); //curl_setopt($ch, CURLOPT_HEADER, true);//jika ingin mengambil info header curl_setopt($ch, CURLOPT_MAXREDIRS, 10);//maksimum redirect curl_setopt($ch, CURLOPT_USERAGENT, "Mozilla/5.0 (Windows NT 6.1; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/37.0.2062.120 Safari/537.36"); curl_setopt($ch, CURLOPT_POST, 1); curl_setopt($ch, CURLOPT_POSTFIELDS,http_build_query($postdata,'','&')); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false); // allow https verification if true curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, false); // check common name and verify with host name curl_setopt($ch, CURLOPT_CONNECTTIMEOUT ,0); //The number of seconds to wait while trying to connect. Use 0 to wait indefinitely. curl_setopt($ch, CURLOPT_TIMEOUT, 40); //timeout in seconds $response = curl_exec ($ch); $http_status = curl_getinfo($ch, CURLINFO_HTTP_CODE); $curl_error = curl_error($ch); curl_close ($ch);
PHP CURL post gzip
$ch = curl_init(); curl_setopt($ch, CURLOPT_URL,$url); curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, 0); curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, 0); curl_setopt($ch, CURLOPT_RETURNTRANSFER,1); curl_setopt($ch, CURLOPT_REFERER, "http://google.com" ); curl_setopt($ch, CURLOPT_FOLLOWLOCATION, TRUE); $header = array( 'Connection: keep-alive', 'Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8', 'Upgrade-Insecure-Requests: 1', 'User-Agent: Mozilla/5.0 (Windows NT 6.1; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/45.0.2454.85 Safari/537.36', 'Accept-Encoding: gzip, deflate, sdch', 'Accept-Language: id-ID,id;q=0.8,en-US;q=0.6,en;q=0.4' ); curl_setopt($ch, CURLOPT_HTTPHEADER, $header); curl_setopt($ch,CURLOPT_ENCODING , "gzip"); //curl_setopt($ch, CURLOPT_COOKIEJAR, $file_cookie);//jika menggunakan cookie //curl_setopt($ch, CURLOPT_COOKIEFILE, $file_cookie);//jika menggunakan cookie curl_setopt($ch, CURLOPT_TIMEOUT, 30); $info = curl_exec($ch); $http_status = curl_getinfo($ch, CURLINFO_HTTP_CODE); $curl_error = curl_error($ch); curl_close($ch);
PHP CURL post json
$data = array("name" => "Hagrid", "age" => "36"); $data_string = json_encode($data); $ch = curl_init($url); curl_setopt($ch, CURLOPT_CUSTOMREQUEST, "POST"); curl_setopt($ch, CURLOPT_POSTFIELDS, $data_string); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_HTTPHEADER, array( 'Content-Type: application/json', 'Content-Length: ' . strlen($data_string)) ); curl_setopt($ch, CURLOPT_REFERER, 'http://www.example.com/1'); $result = curl_exec($ch); $http_status = curl_getinfo($ch, CURLINFO_HTTP_CODE); $curl_error = curl_error($ch); curl_close ($ch);
Penanganan di file tujuannya adalah sebagai berikut, proses-json.php:
<?php //untuk test menerima post dengan curl json $jsonPost = file_get_contents("php://input"); $arrayPost = json_decode($jsonPost, true); echo '<pre>'; print_r($arrayPost); echo '</pre>'; echo '<pre>'; print_r($_SERVER); echo '</pre>'; echo '<pre>'; print_r($_POST); echo '</pre>';
PHP CURL dengan www authenticate
<?php $headers = array(); $headers[] = 'WWW-Authenticate: 0809contohkeyanda389329'; $headers[] = 'Connection: keep-alive'; $postdata = array('param1'=>'value1'); $ch = curl_init(); curl_setopt($ch, CURLOPT_URL,$url); curl_setopt($ch, CURLOPT_HTTPHEADER, $headers); curl_setopt($ch, CURLOPT_POST, 1); curl_setopt($ch, CURLOPT_POSTFIELDS,http_build_query($postdata,'','&')); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false); curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, 0); curl_setopt($ch, CURLOPT_TIMEOUT, 40); curl_setopt($ch, CURLINFO_HEADER_OUT, 1); $result = curl_exec ($ch); $http_status = curl_getinfo($ch, CURLINFO_HTTP_CODE); $curl_error = curl_error($ch); curl_close ($ch);
Di server tujuannya, misal proses-authen.php
<?php $authenticate = isset($_SERVER['HTTP_WWW_AUTHENTICATE']) ? $_SERVER['HTTP_WWW_AUTHENTICATE'] : ''; if (empty($authenticate)) { echo 'tidak ada authenticate'; }
PHP CURL authenticate dengan user dan password
$ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $url); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_USERPWD, "$username:$password"); curl_setopt($ch, CURLOPT_HTTPAUTH, CURLAUTH_BASIC); $output = curl_exec($ch); $info = curl_getinfo($ch); curl_close($ch);
PHP CURL menggunakan proxy dan user passwordnya
$proxy = '111.1.1.1:8888'; $proxyauth = 'user:password'; $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $url); curl_setopt($ch, CURLOPT_PROXY, $proxy); curl_setopt($ch, CURLOPT_PROXYUSERPWD, $proxyauth); //curl_setopt($ch, CURLOPT_PROXYTYPE, CURLPROXY_SOCKS5); curl_setopt($ch, CURLOPT_FOLLOWLOCATION, 1) curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); $response = curl_exec($ch); curl_close($ch);
PHP CURL upload multiple file ke server
Sudah pernah diposting di artikel sebelumnya, silahkan klik disini.
Demikian, semoga bermanfaat.