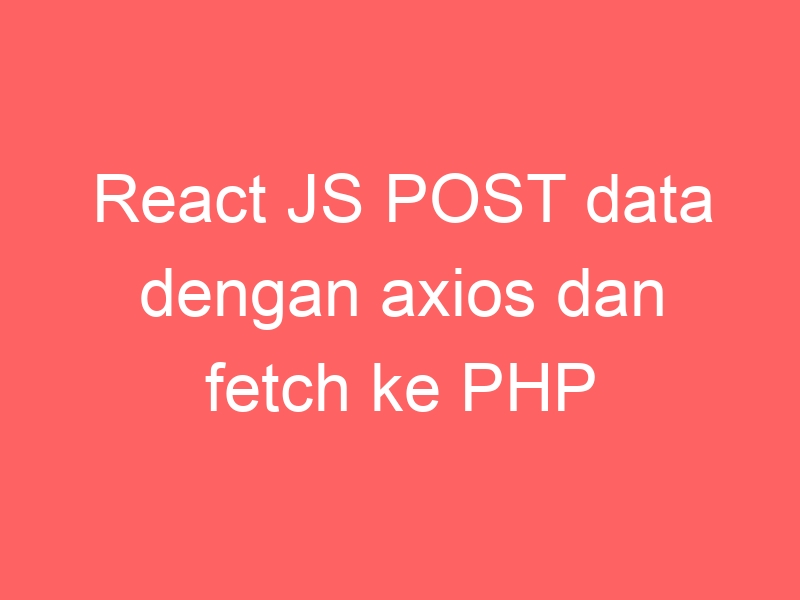
Bismillaahirrohmaanirrohiim…
Berikut ini adalah contoh penggunaan axios dan fetch pada react JS untuk post data ke server yang menggunakan file PHP.
Yang perlu diinstall untuk menggunakan contoh ini adalah:
npm install axios qs
Antara axios dan fetch tentu mempunyai kelebihan dan kekurangan masing-masing.
Seorang programmer bebas memilih mana yang cocok dan terbaik sesuai kebutuhannya.
– Axios Menggunakan submit form ke PHP
Di sini seluruh input pada form langsung didefinisikan pada variabel
const data = new FormData(e.target);
Axios const data = new FormData(e.target); data.append('addparam','addvalue'); axios.post('http://url.com/path.php', data) .then(function(response) { //success console.log(response); }) .catch(function (error) { console.log(error); }) .finally(function(){ console.log('finish') }) PHP $json = json_encode($_POST);
– Axios Menggunakan parameter key value ke PHP
Cara ini digunakan untuk mendefinisikan parameter satu persatu, biasanya dipakai untuk validasi, dll
penanganan dengan PHP nya juga menggunakan perintah
$json = file_get_contents('php://input');
Axios axios.post('http://url.com/path.php', { param1: 'value 1', name: this.state.name, age: this.state.age, }) .then(function(response) { //success console.log(response); }) .catch(function (error) { console.log(error); }) .finally(function(){ console.log('finish') }) PHP $json = file_get_contents('php://input');
– Axios Menggunakan parameter key value ke PHP dengan query string
Sama seperti cara di atas, bedanya penanganan datanya di PHP menggunakan
$json = json_encode($_POST);
Axios import qs from "qs";//query string const exp = { titre: this.state.user_nama, contenu: this.state.user_email, datePub: 'pub' , userID: 1 }; axios.post('http://url.com/path.php', qs.stringify(exp)) .then(function(response) { console.log(response); }) .catch(function (error) { console.log('err: '+error); }) .finally(function(){ console.log('sukses semua') }) PHP $json = json_encode($_POST);
– Fetch Menggunakan submit form ke PHP
Di sini seluruh input pada form langsung didefinisikan pada variabel
const data = new FormData(e.target);
Fetch const data = new FormData(e.target); fetch('http://url.com/path.php', { method: 'POST', body: data, }) .then(function (response) { return response.json(); }) .then(function (result) { console.log(JSON.stringify(result)); }) .catch (function (error) { console.log('Request failed', error); }); PHP $json = json_encode($_POST);
– Fetch Menggunakan parameter key value ke PHP
Cara ini digunakan untuk mendefinisikan parameter satu persatu, biasanya dipakai untuk validasi, dll
penanganan dengan PHP nya sama menggunakan perintah
$json = json_encode($_POST);
Fetch import qs from "qs";//query string const exp = { titre: this.state.user_nama, contenu: this.state.user_email, datePub: 'pub' , userID: 1 }; fetch('http://url.com/path.php', { method: 'POST', headers: {'Content-Type':'application/x-www-form-urlencoded'}, body: qs.stringify(exp), }) .then(function (response) { return response.json(); }) .then(function (result) { console.log(JSON.stringify(result)); }) .catch (function (error) { console.log('Request failed', error); }); PHP $json = json_encode($_POST);
– Axios form upload file ke PHP
Di sini seluruh input pada form langsung didefinisikan pada variabel
const data = new FormData(e.target);
ada tambahan headers jika dipakai untuk upload file.
Axios const cfg = { headers: { 'content-type':'multipart/form-data' } }; axios.post('http://url.com/path.php', data, cfg) .then(function (response) { console.log(response); }) .catch(function (error) { console.log('err: ' + error); }) .finally(function () { console.log('finish') }) PHP $foto = isset($_FILES['foto']) ? $_FILES['foto'] : array(); $foto_name = isset($foto['name']) ? $foto['name'] : ''; if (!empty($foto_name)) { @move_uploaded_file($foto["tmp_name"], $foto_name); } $json = json_encode($_POST);
Demikian catatan singkat ini, semoga bermanfaat.